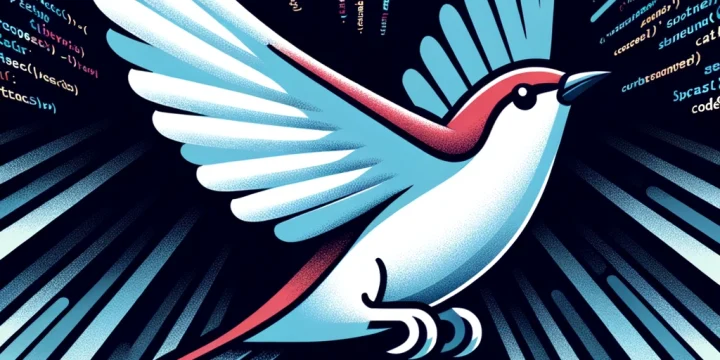
iOS Camera Image Processing and Pose Estimation: A Comprehensive Guide
iOS Camera Image Processing and Pose Estimation With the advent of mobile technology, smartphones have become more powerful and feature-rich than ever before. One of the powerful features of smartphones is their built-in camera. In this article, we will explore how to use the camera in iOS devices to process images and perform pose estimation. Capturing Images with the Camera iOS provides a built-in framework called AVFoundation that allows us to interact with the camera. To capture images, we can make use of the AVCaptureSession class. Here's an example on how to set up a basic AVCaptureSession to capture images: import AVFoundation let session = AVCaptureSession() guard let device = AVCaptureDevice.default(for: .video), let input = try? AVCaptureDeviceInput(device: device) else { // Handle error cases return } session.addInput(input) let output =…